How to Create a Chat App in Android
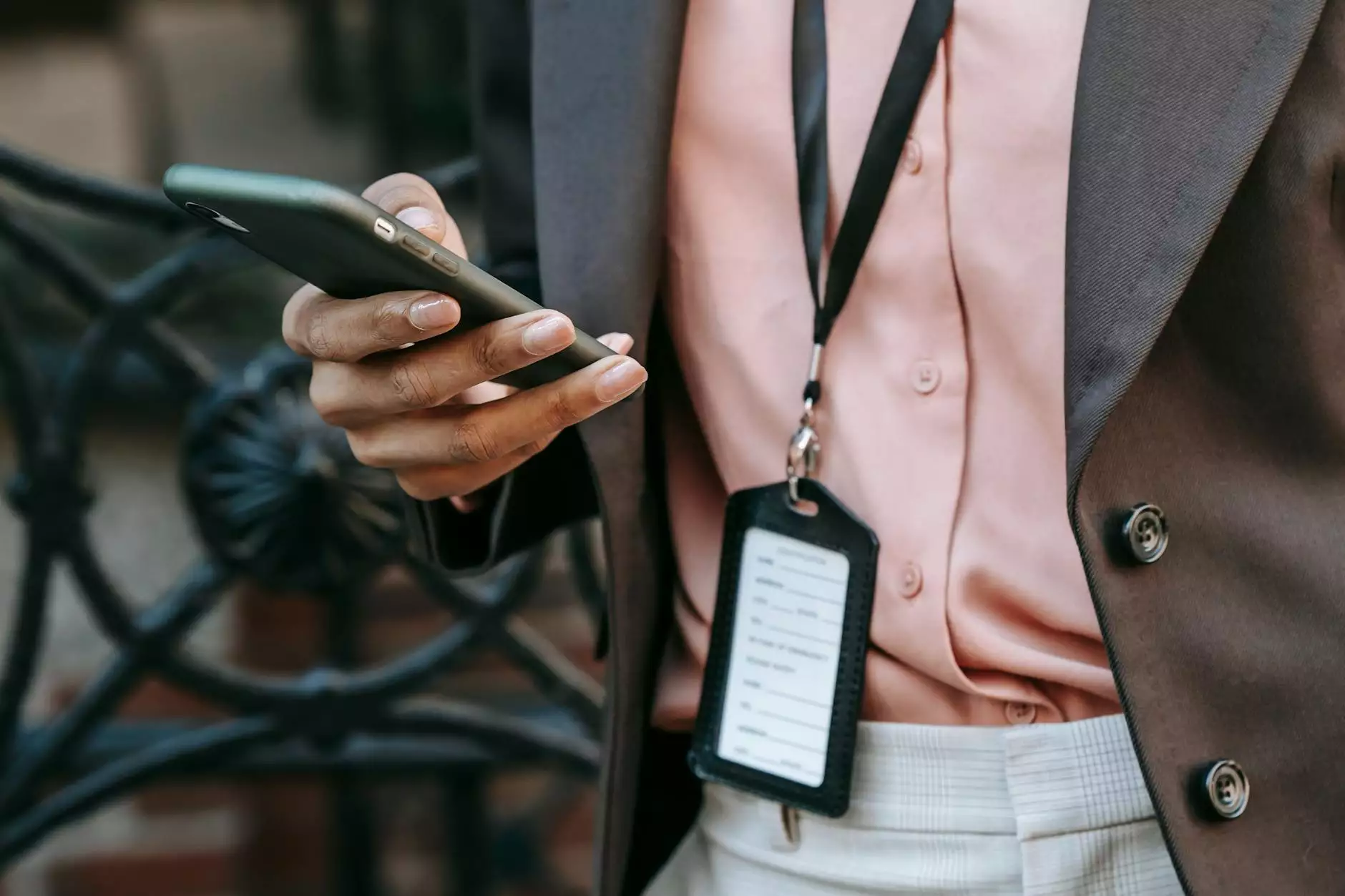
Creating a chat app in Android is an exciting endeavor that not only enhances your programming skills but also provides a platform for real-time communication. In this comprehensive guide, we will delve deep into how to create a chat app in Android, covering everything from the technologies you will use, to design considerations and the essential features that will set your application apart. Let's embark on this journey to develop a remarkable chat application!
1. Understanding the Basics of Chat Applications
Before diving into the technical aspects, it's crucial to understand what a chat app entails. Chat applications typically allow users to send and receive text messages in real time, and they often include additional features such as multimedia sharing, voice messages, and contact management.
The core components of a chat app include:
- User Authentication: Users need to create an account or log in to chat.
- Data Storage: To save messages and user information.
- Real-Time Messaging: To ensure messages are delivered instantly.
- User Interface: A well-designed interface to enhance user experience.
2. Choosing the Right Technology Stack
When learning how to create a chat app in Android, it's essential to select the right technologies that will support your app's features and functionality. Here’s a typical stack you might consider:
- Programming Language: Kotlin or Java - Kotlin is recommended for new Android projects due to its modern features.
- Backend Service: Firebase, Socket.io, or custom REST API - Firebase is popular due to its seamless integration and real-time capabilities.
- Database: Firestore (for Firebase), SQLite, or Room for local storage.
- Networking Library: Retrofit or Volley for HTTP requests.
- User Interface Framework: XML layout in Android or Jetpack Compose for modern UI design.
3. Setting Up Your Development Environment
To start building your chat app, you need to set up your development environment:
- Install Android Studio: The official integrated development environment (IDE) for Android app development.
- SDK and Emulators: Ensure you have the latest Android SDK and set up emulators for testing.
- Version Control System: Use Git for version control to manage changes in your codebase efficiently.
4. Implementing User Authentication
User authentication is the first step in making a secure chat application. With Firebase Authentication, you can easily implement various authentication methods including email/password, Google Sign-In, and more.
Steps to implement authentication using Firebase:
- Create a project in the Firebase Console.
- Add your Android app to the project and download the google-services.json file.
- Add Firebase Authentication dependencies to your project.
- Set up the authentication methods in the Firebase Console.
- Implement the sign-in and sign-up functionalities in your app.
5. Building the Chat Interface
The user interface is a critical aspect of your chat application. The design should be intuitive and user-friendly.
Here’s how to design your chat interface:
- Chat List: Create a RecyclerView to display the list of chats.
- Message Input Box: Use EditText for message input, with a Send button.
- Message Display: Design message bubbles for incoming and outgoing messages.
Example XML Layout for Chat Interface
6. Implementing Real-Time Messaging
For your chat app to function as a real-time messaging platform, you must implement a solution that allows immediate message delivery. Using Firebase Cloud Firestore enables real-time updates, but you can also use WebSocket for a more customizable approach.
Here’s how to use Firebase for real-time messaging:
- Create a Firestore collection for messages.
- Insert new messages into the collection as they are sent.
- Listen for changes to the collection to display incoming messages in real time.
Example Firestore Listener Implementation
Firestore db = FirebaseFirestore.getInstance(); db.collection("messages").addSnapshotListener(new EventListener() { @Override public void onEvent(QuerySnapshot value, FirebaseFirestoreException e) { if (e != null) { Log.w(TAG, "Listen failed.", e); return; } // Process the messages here } });7. Storing and Retrieving Messages
Efficient data management is crucial for any chat application. Ensure messages are stored securely and organized logically in your database.
You’ll want to consider the following:
- Message Structure: Design your message data model to include fields such as senderID, receiverID, timestamp, and message content.
- Querying Messages: Implement pagination or lazy loading to retrieve messages in batches.
- Handling Offline Capability: Use Firestore’s offline persistence feature to allow users to send and receive messages without an internet connection.
8. Adding Multimedia Support
Modern chat applications often provide users the ability to share images, videos, and audio. You can utilize Firebase Storage for storing multimedia files.
Steps to add multimedia support:
- Integrate Firebase Storage into your project.
- Provide users with an option to upload media through the chat interface.
- Store the media URL in your Firestore messages collection when a file is successfully uploaded.
9. Enhancing User Experience with Notifications
Push notifications are vital for informing users of incoming messages—especially when they are not actively using the app. Firebase Cloud Messaging (FCM) makes this implementation straightforward.
- Set up Firebase Cloud Messaging in your project.
- Implement notification handling code in your app.
- Trigger notifications for incoming messages using FCM.
10. Ensuring Security and Privacy
Security should be a top priority when creating a chat application. Here are important practices to follow:
- Data Encryption: Ensure all messages are encrypted both in transit and at rest.
- Secure Authentication: Use strong password policies and consider implementing multi-factor authentication.
- User Privacy: Provide users with privacy settings to control who can contact them or view their profiles.
11. Testing Your Chat Application
Before releasing your app to the public, comprehensive testing is essential. Perform the following tests:
- Unit Testing: Ensure each component behaves as expected.
- Integration Testing: Test how components work together, particularly the back-end and front-end.
- User Acceptance Testing: Allow real users to test your app and gather feedback.
12. Deploying Your Chat Application
Once testing is complete, you are ready to deploy your chat app:
- Prepare a production version of your app.
- Upload the APK to the Google Play Store.
- Promote your app through social channels to attract users.
13. Continuous Improvement and Maintenance
The launch of your app is just the beginning. To keep it relevant and functional:
- Monitor User Feedback: Pay attention to user reviews and ratings for potential improvements.
- Regular Updates: Implement new features and fix bugs regularly to enhance user satisfaction.
- Analyze User Data: Use analytics to understand user behavior and optimize app performance.
Conclusion
Building a chat application for Android can be a rewarding project that hones your development skills and offers valuable experience in mobile app development. By following the above steps on how to create a chat app in Android, you can develop a robust and feature-rich chatting platform that meets users' needs. Always remember to focus on the user experience, maintain security, and keep iterating on your app based on user feedback for the best results.
For more information and resources on mobile app development, visit nandbox.com.